Image
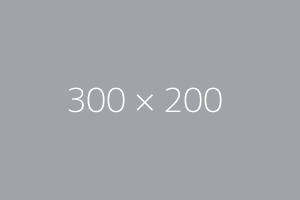
import React from 'react';
import { Image } from 'seduo-ui';
const Example = () => (
<Image
src="https://dummyimage.com/300x200/9fa4a8/fff.png"
width="300"
height="200"
alt="Martin Basl, Livesport, s.r.o., Business Development Manager"
/>
);
export default Example;
Image with overlay composition
Image overlay creates a layer above the image where any element can be placed in each corner.
import React from 'react';
import { Image, ImageOverlay } from 'seduo-ui';
const Square = () => <div style={{ width: '24px', height: '24px', background: 'black' }} />;
const Example = () => (
<div style={{ width: '300px' }}>
<ImageOverlay topLeft={<Square />} topRight={<Square />} bottomLeft={<Square />} bottomRight={<Square />}>
<Image src="https://dummyimage.com/300x200/9fa4a8/fff.png" alt="Image title" />
</ImageOverlay>
</div>
);
export default Example;
RoundedImage
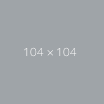
import React from 'react';
import { Image } from 'seduo-ui';
const Example = () => (
<Image
src="https://dummyimage.com/104x104/9fa4a8/fff.png"
width="104px"
height="104px"
className="rounded-circle"
alt="Martin Basl, Livesport, s.r.o., Business Development Manager"
/>
);
export default Example;
RoundedImage with circle around
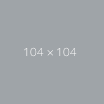
import React from 'react';
import { Image } from 'seduo-ui';
const Example = () => (
<div className="rounded-circle-around">
<Image
src="https://dummyimage.com/104x104/9fa4a8/fff.png"
width="104"
height="104"
className="rounded-circle"
alt="Martin Basl, Livesport, s.r.o., Business Development Manager"
/>
</div>
);
export default Example;